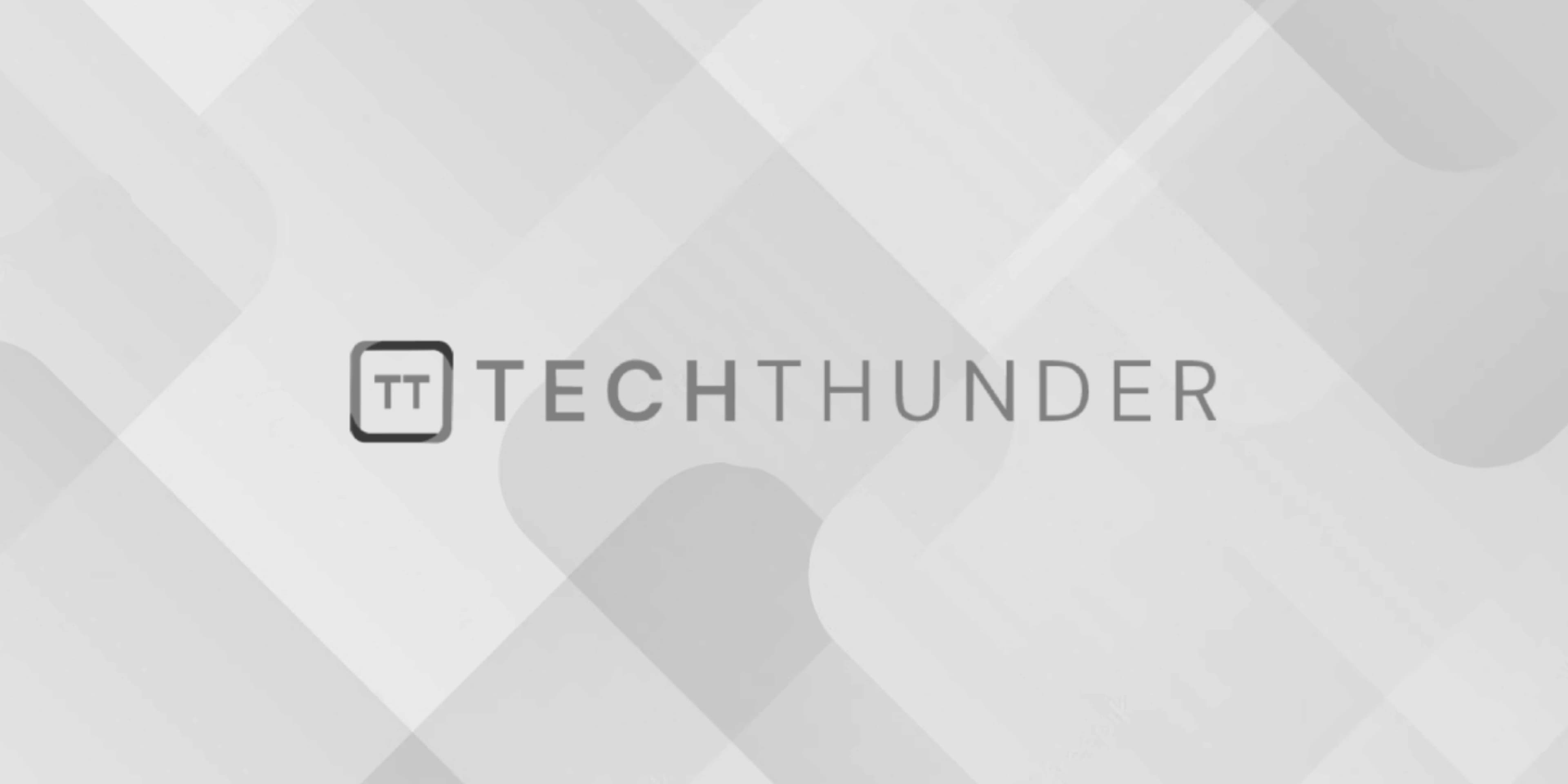
147 views
Sum of digits in C++
To calculate the sum of digits of an integer in C++, you can use a loop to extract each digit and add it to a running sum. Here’s a simple example:
C++
#include <iostream>
int main() {
int number, digit, sum = 0;
// Input an integer
std::cout << "Enter an integer: ";
std::cin >> number;
// Ensure the number is non-negative
number = abs(number);
// Calculate the sum of digits
while (number > 0) {
digit = number % 10; // Get the last digit
sum += digit; // Add the digit to the sum
number /= 10; // Remove the last digit
}
std::cout << "Sum of digits: " << sum << std::endl;
return 0;
}
In this program:
- The user is prompted to enter an integer.
- The
abs
function is used to ensure that the number is non-negative, as the sum of digits doesn’t depend on the sign. - A
while
loop is used to extract each digit from the number. The last digit is obtained using the modulo operator (% 10
), added to thesum
variable, and then removed from the number using integer division (/ 10
). - The
sum
variable keeps track of the sum of digits. - The program displays the sum of digits as the output.
Compile and run this program, and it will calculate and display the sum of the digits of the entered integer.