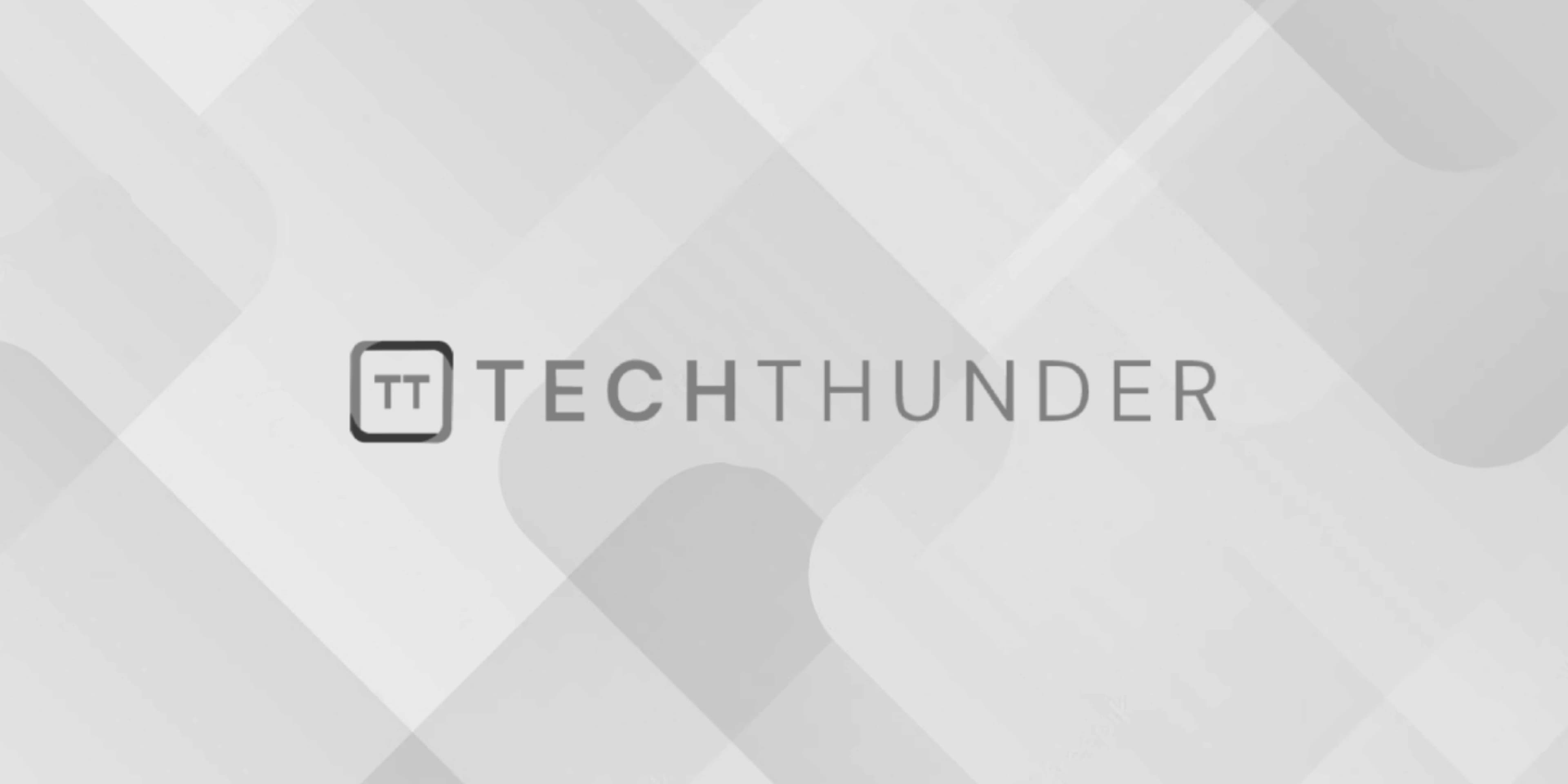
Const keyword in C++
The C++ const
keyword is used to indicate that an entity (such as a variable, pointer, or member function) is constant, meaning its value or behavior cannot be modified after it has been initialized or defined. The const
keyword is a fundamental feature of C++ that plays a crucial role in enforcing data immutability, enhancing code safety, and enabling compiler optimizations.
Here are some common uses of the const
keyword in C++:
- Constant Variables:
const int max_size = 100; // Declaring a constant integer variable
A constant variable cannot be modified once it’s assigned a value. Attempts to modify it will result in a compilation error.
- Constant Pointers:
const int* ptr; // Pointer to a constant integer
int const* ptr; // Equivalent declaration
A constant pointer can be used to point to a constant variable. It means you can’t modify the value through this pointer, but you can change the pointer to point to something else.
- Pointer to a Constant:
int value = 42;
const int* ptr = &value; // Pointer to a constant integer
A pointer to a constant can’t be used to modify the value it points to, but it can be changed to point to another constant integer.
- Constant Member Functions:
class MyClass {
public:
void NonConstMethod() {
// This method can modify the object's data members.
}
void ConstMethod() const {
// This method cannot modify the object's data members.
}
};
A const
member function is a promise that it won’t modify the object’s data members. It can be called on constant objects and prevents unintended modifications.
- Constant Member Variables:
class MyClass {
public:
const int max_value = 100; // Constant member variable
};
A constant member variable is a data member of a class that cannot be modified for any instance of the class. It’s often used for configuration values that should be constant across all objects of the class.
- Constant References:
const int& ref = some_integer; // Constant reference
A constant reference allows you to refer to a value without allowing it to be modified through the reference.
The const
keyword is essential for code clarity, maintainability, and safety. It helps prevent accidental modifications of data, ensures that certain functions don’t have side effects, and allows the compiler to perform optimizations based on the immutability of data.