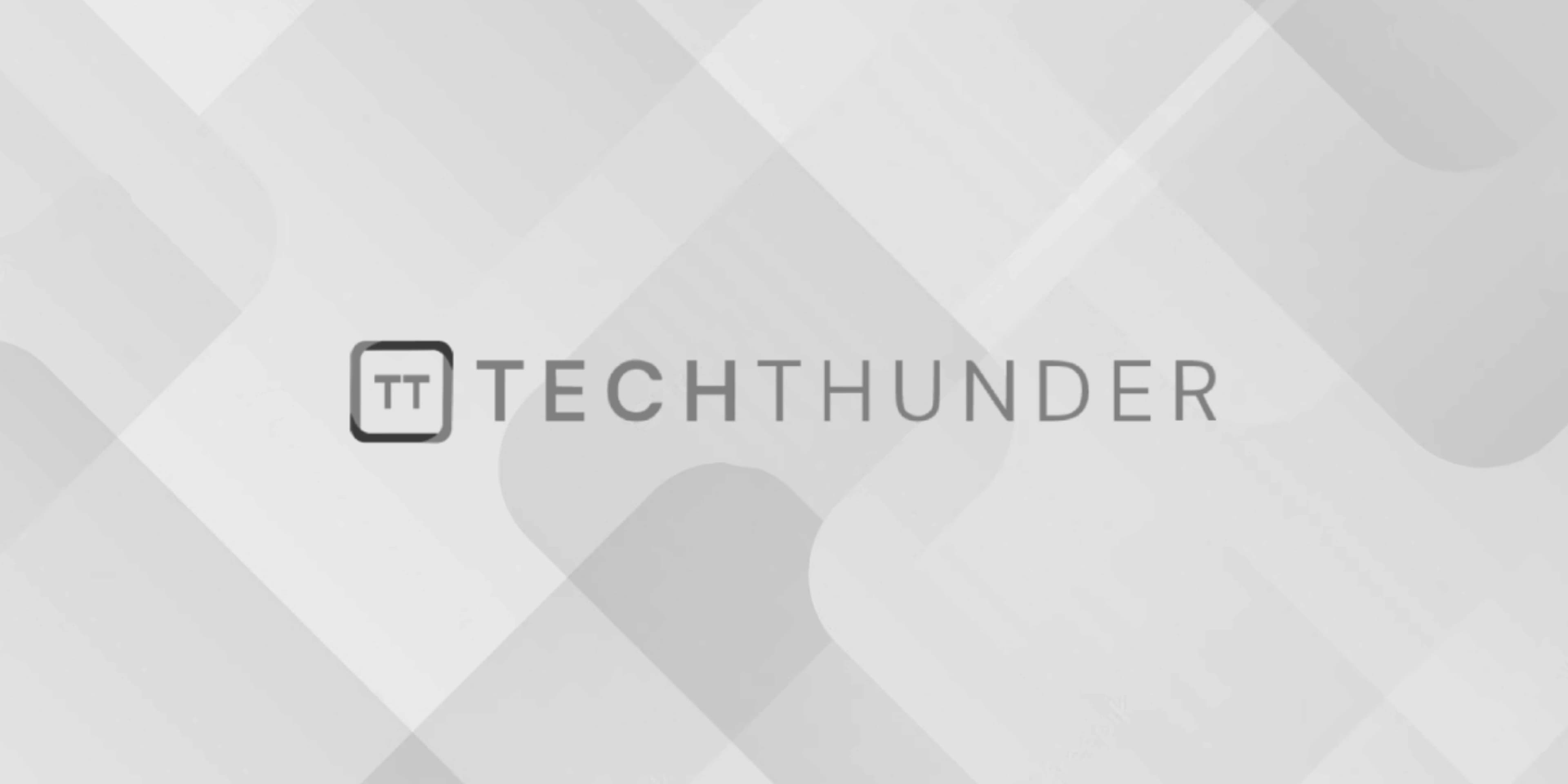
248 views
Naked Function Calls in C++
The C++ naked function call typically refers to a function call made without any reference to an object or a class. It means calling a standalone function rather than a member function of a class or an object’s member function. Naked function calls are a common practice when invoking free functions, global functions, or static member functions.
Here are a few examples to illustrate naked function calls in C++:
- Global Functions: A global function is a function declared outside any class or namespace. To call a global function, you simply use the function’s name followed by its arguments.
C++
#include <iostream>
// Global function
void printMessage(const std::string& message) {
std::cout << message << std::endl;
}
int main() {
printMessage("Hello, world!"); // Naked function call
return 0;
}
- Static Member Functions: In a class, static member functions belong to the class rather than an instance of the class. They can be called using the class name and the scope resolution operator
::
.
C++
#include <iostream>
class Math {
public:
static int add(int a, int b) {
return a + b;
}
};
int main() {
int sum = Math::add(5, 3); // Naked function call to a static member function
std::cout << "Sum: " << sum << std::endl;
return 0;
}
- Free Functions: Free functions are functions that are not part of a class or namespace. You can call them directly by their name.
C++
#include <iostream>
// Free function
int multiply(int a, int b) {
return a * b;
}
int main() {
int result = multiply(4, 6); // Naked function call to a free function
std::cout << "Result: " << result << std::endl;
return 0;
}
Naked function calls are essential for invoking functions that are not associated with any specific object or class instance. They are straightforward to use and are a fundamental part of C++ programming, especially when working with global functions, static member functions, and free functions.