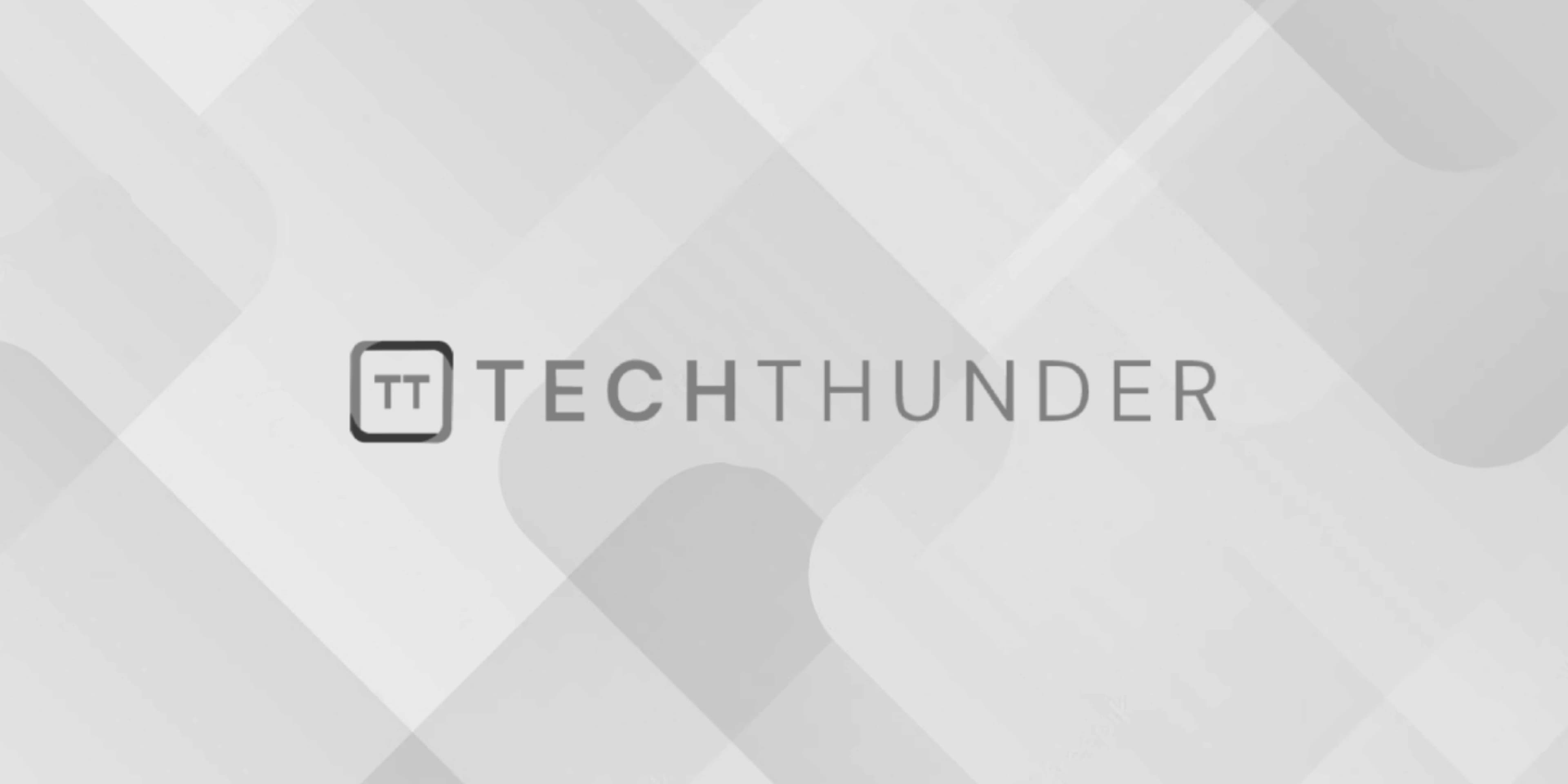
Fibonacci Series in C++
The Fibonacci series is a sequence of numbers where each number is the sum of the two preceding ones. It usually starts with 0 and 1. Here’s how to generate the Fibonacci series in C++ using different methods:
Method 1: Using a Loop
#include <iostream>
int main() {
int n;
long long int a = 0, b = 1, nextTerm;
std::cout << "Enter the number of terms: ";
std::cin >> n;
std::cout << "Fibonacci Series: ";
for (int i = 1; i <= n; ++i) {
if (i == 1) {
std::cout << a << " ";
continue;
}
if (i == 2) {
std::cout << b << " ";
continue;
}
nextTerm = a + b;
a = b;
b = nextTerm;
std::cout << nextTerm << " ";
}
return 0;
}
Method 2: Using Recursion
#include <iostream>
long long int fibonacci(int n) {
if (n <= 0) {
return 0;
} else if (n == 1) {
return 1;
} else {
return fibonacci(n - 1) + fibonacci(n - 2);
}
}
int main() {
int n;
std::cout << "Enter the number of terms: ";
std::cin >> n;
std::cout << "Fibonacci Series: ";
for (int i = 0; i < n; ++i) {
std::cout << fibonacci(i) << " ";
}
return 0;
}
In Method 1, a for
loop is used to generate the Fibonacci series iteratively. It starts with a
and b
initialized to 0 and 1, and then calculates and prints each subsequent term in the series.
In Method 2, a recursive function fibonacci
is used to generate the Fibonacci series. The function returns the nth term in the series. The main
function calls this function for each term in the series.
You can choose the method that suits your needs. Method 1 is more efficient for large values of n
as it avoids recomputation, while Method 2 is a straightforward recursive approach.