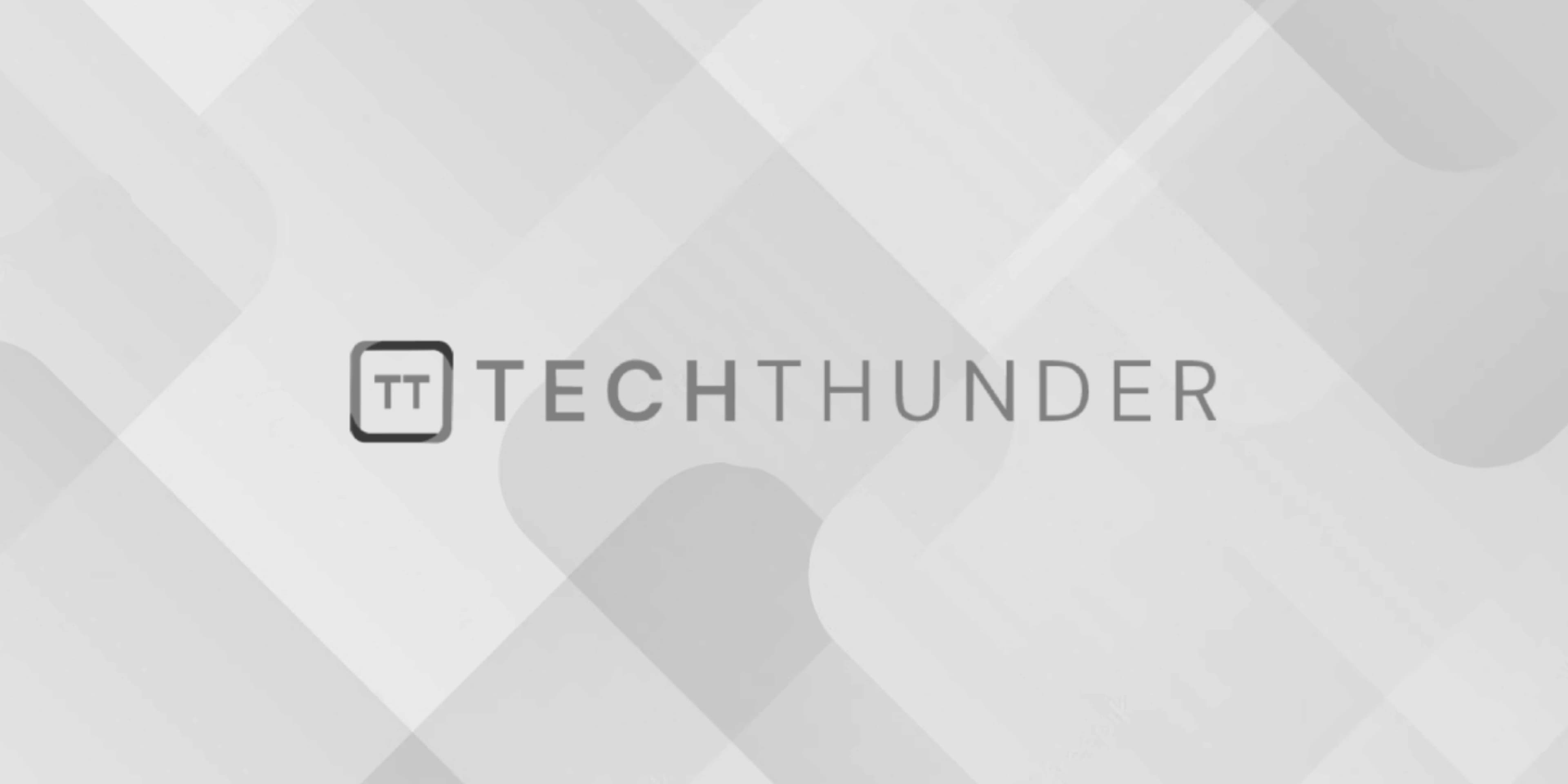
221 views
Binary Decision Tree in C++
A binary decision tree is a tree-like data structure in which each node has at most two children, and it is often used in decision-making processes, such as in computer science algorithms, machine learning, and game theory. In C++, you can implement a binary decision tree using a custom class.
Here’s a simple example of how to implement a binary decision tree:
C++
#include <iostream>
// Node structure for the binary decision tree
struct Node {
std::string question;
Node* yesNode;
Node* noNode;
Node(const std::string& q) : question(q), yesNode(nullptr), noNode(nullptr) {}
};
// Function to traverse the decision tree
void traverse(Node* currentNode) {
if (currentNode == nullptr) {
return;
}
std::cout << currentNode->question << " (yes/no): ";
std::string response;
std::cin >> response;
if (response == "yes" && currentNode->yesNode != nullptr) {
traverse(currentNode->yesNode);
} else if (response == "no" && currentNode->noNode != nullptr) {
traverse(currentNode->noNode);
} else {
std::cout << "Invalid response. Exiting." << std::endl;
}
}
int main() {
Node* root = new Node("Is it an animal?");
root->yesNode = new Node("Does it have fur?");
root->noNode = new Node("Is it a mineral?");
root->yesNode->yesNode = new Node("It might be a mammal.");
root->yesNode->noNode = new Node("It might be a bird.");
root->noNode->yesNode = new Node("It's probably a rock.");
root->noNode->noNode = new Node("It's probably not a rock.");
std::cout << "Welcome to the Binary Decision Tree!" << std::endl;
traverse(root);
// Deallocate memory
delete root->yesNode->yesNode;
delete root->yesNode->noNode;
delete root->noNode->yesNode;
delete root->noNode->noNode;
delete root->yesNode;
delete root->noNode;
delete root;
return 0;
}
In this example:
- We define a
Node
structure to represent each node in the decision tree. Each node has a question, a “yes” child, and a “no” child. - We create a simple decision tree with a few questions and answers.
- The
traverse
function allows the user to navigate through the decision tree by answering “yes” or “no” to each question. - The program starts at the root of the tree and traverses down to the leaves, making decisions based on user responses.
- We deallocate memory to avoid memory leaks when the program exits.
This example is a basic demonstration of how to implement a binary decision tree. You can extend and customize it for more complex decision-making scenarios.