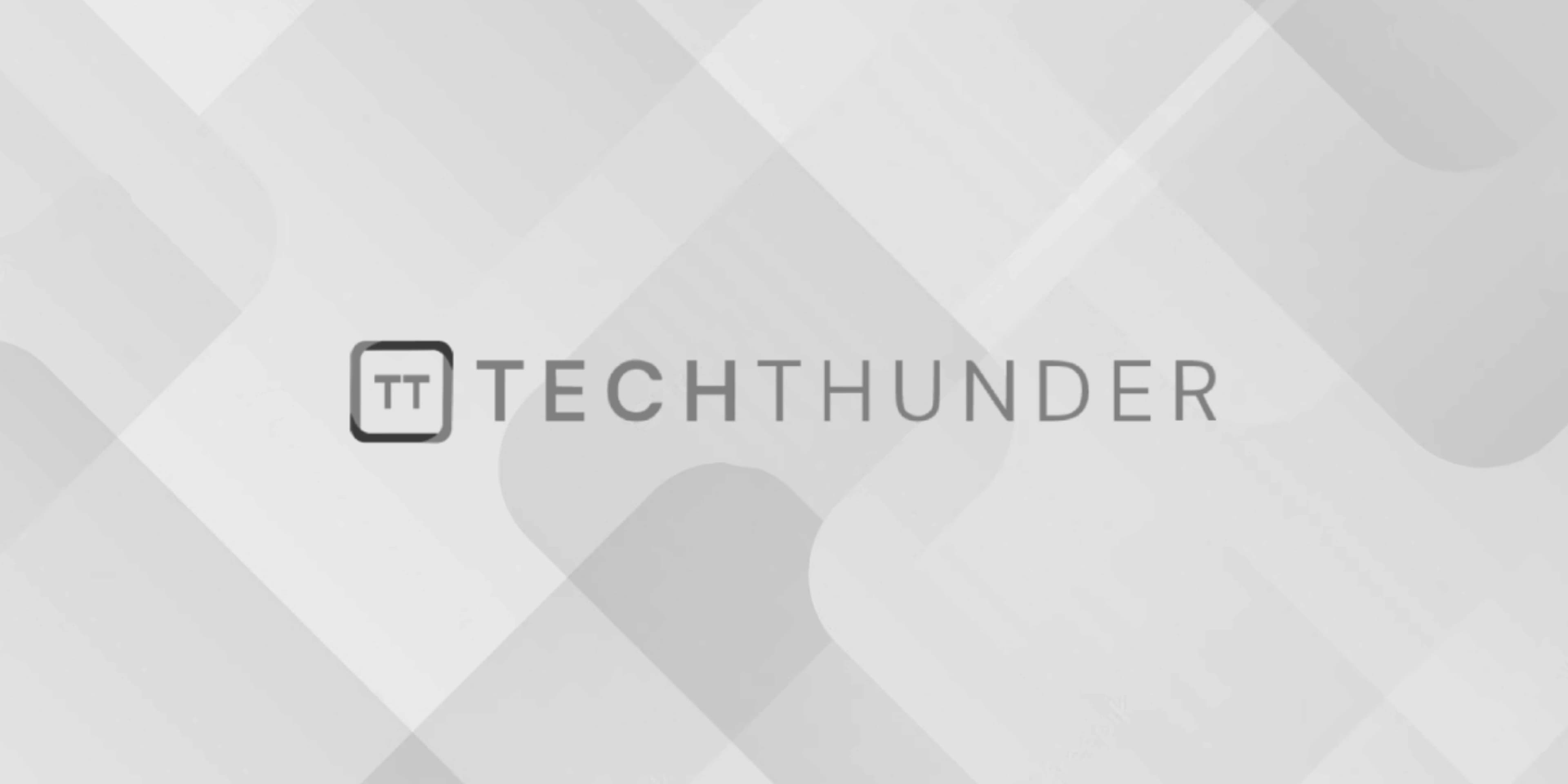
139 views
Student Data Management in C++
Managing student data in C++ involves creating a program that can store, retrieve, and manipulate information about students. You can use data structures like classes, arrays, vectors, and files to achieve this. Here’s a basic example of student data management in C++:
C++
#include <iostream>
#include <fstream>
#include <vector>
class Student {
public:
std::string name;
int rollNumber;
double grade;
// Constructor to initialize student data
Student(std::string n, int roll, double g) : name(n), rollNumber(roll), grade(g) {}
// Display student information
void display() {
std::cout << "Name: " << name << std::endl;
std::cout << "Roll Number: " << rollNumber << std::endl;
std::cout << "Grade: " << grade << std::endl;
}
};
// Function to add a new student to the database
void addStudent(std::vector<Student>& students) {
std::string name;
int rollNumber;
double grade;
std::cout << "Enter student name: ";
std::cin >> name;
std::cout << "Enter roll number: ";
std::cin >> rollNumber;
std::cout << "Enter grade: ";
std::cin >> grade;
students.emplace_back(name, rollNumber, grade);
}
// Function to display all student data
void displayAllStudents(const std::vector<Student>& students) {
std::cout << "Student Data:" << std::endl;
for (const Student& student : students) {
student.display();
std::cout << std::endl;
}
}
// Function to save student data to a file
void saveStudentData(const std::vector<Student>& students, const std::string& filename) {
std::ofstream file(filename);
if (!file.is_open()) {
std::cerr << "Error opening file for writing." << std::endl;
return;
}
for (const Student& student : students) {
file << student.name << " " << student.rollNumber << " " << student.grade << std::endl;
}
file.close();
std::cout << "Student data saved to " << filename << std::endl;
}
int main() {
std::vector<Student> students;
std::string filename = "student_data.txt";
// Load student data from a file (if available)
std::ifstream file(filename);
if (file.is_open()) {
std::string name;
int rollNumber;
double grade;
while (file >> name >> rollNumber >> grade) {
students.emplace_back(name, rollNumber, grade);
}
file.close();
}
int choice;
do {
std::cout << "Student Data Management System" << std::endl;
std::cout << "1. Add Student" << std::endl;
std::cout << "2. Display All Students" << std::endl;
std::cout << "3. Save Student Data" << std::endl;
std::cout << "4. Quit" << std::endl;
std::cout << "Enter your choice: ";
std::cin >> choice;
switch (choice) {
case 1:
addStudent(students);
break;
case 2:
displayAllStudents(students);
break;
case 3:
saveStudentData(students, filename);
break;
case 4:
std::cout << "Exiting the program." << std::endl;
break;
default:
std::cout << "Invalid choice. Please try again." << std::endl;
}
} while (choice != 4);
return 0;
}
In this example:
- We define a
Student
class to store information about each student, including their name, roll number, and grade. - The program provides options to add a new student, display all students, and save the student data to a file.
- Student data is stored in a
std::vector<Student>
. - Data is loaded from a file (
student_data.txt
) if it exists when the program starts and saved to the same file when the user chooses the save option.
This is a simple example of student data management, and you can extend and customize it to suit your specific requirements, such as adding more student attributes or implementing additional functionalities.