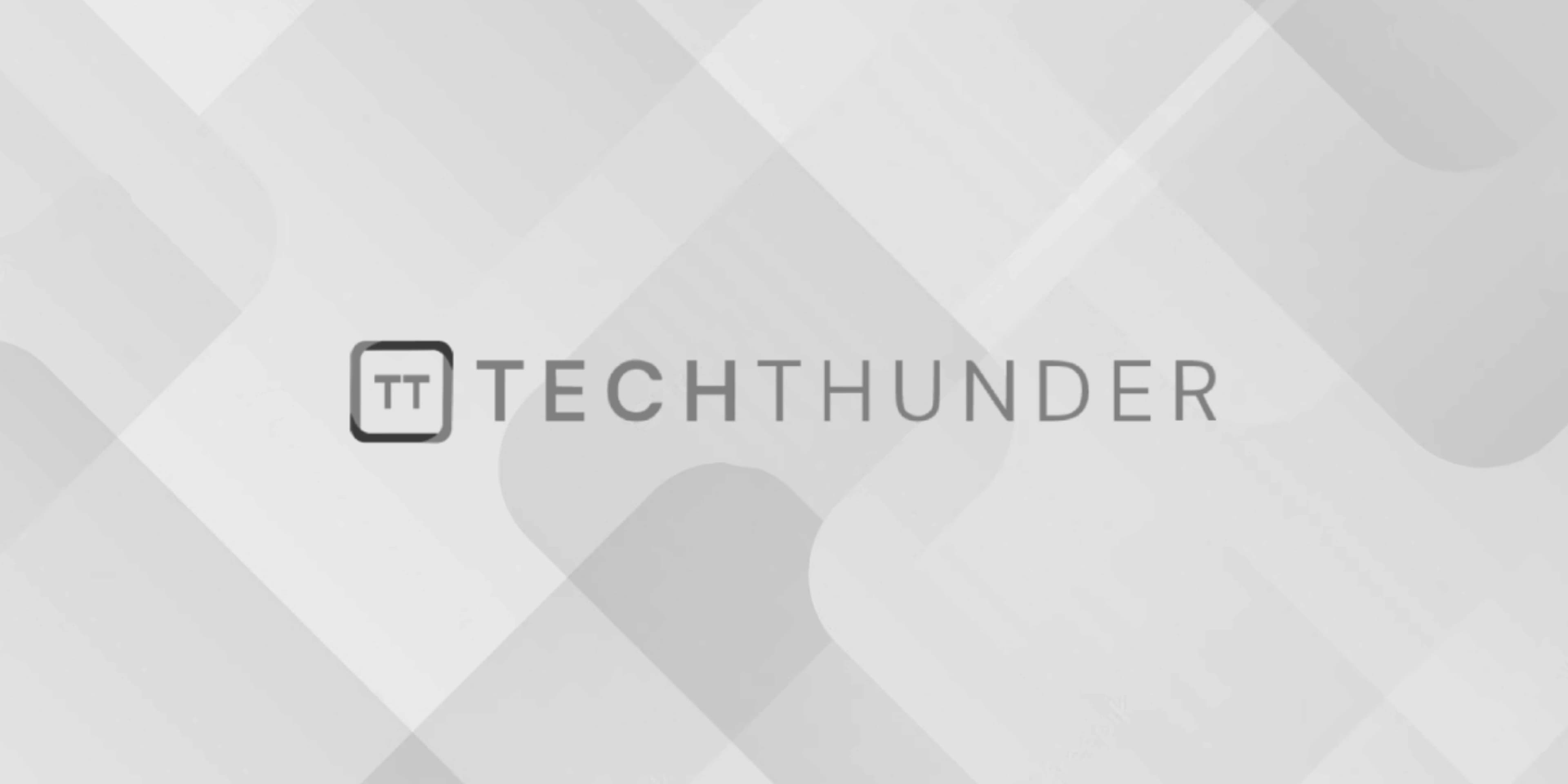
C++ While Loop
The C++ while
loop is a control structure that allows you to repeatedly execute a block of code as long as a specified condition is true. Here’s the basic syntax of a while
loop:
while (condition) {
// Code to be executed repeatedly as long as 'condition' is true
}
Here’s how the while
loop works:
- The
condition
is evaluated first. If it’s true, the code block inside the loop is executed. - After the code block is executed, the
condition
is evaluated again. - If the
condition
is still true, the code block is executed again, and this process continues until thecondition
becomes false. - Once the
condition
becomes false, the loop terminates, and program control moves to the next statement after thewhile
loop.
Here’s a simple example of a while
loop that counts from 1 to 5:
#include <iostream>
int main() {
int i = 1;
while (i <= 5) {
std::cout << i << " ";
i++;
}
std::cout << std::endl;
return 0;
}
In this example, the loop starts with i
equal to 1. The while
loop continues to execute as long as i
is less than or equal to 5. Inside the loop, i
is printed, and then it is incremented by 1 (i++
). The loop continues until i
becomes 6, at which point the condition becomes false, and the loop terminates.
Remember that you need to ensure that the condition in a while
loop eventually becomes false; otherwise, the loop will run indefinitely, causing a program to hang.
You can also use the while
loop with different types of conditions, including conditions that involve user input, calculations, or variables that change during the loop’s execution. The while
loop provides a flexible way to perform repetitive tasks in your C++ programs.