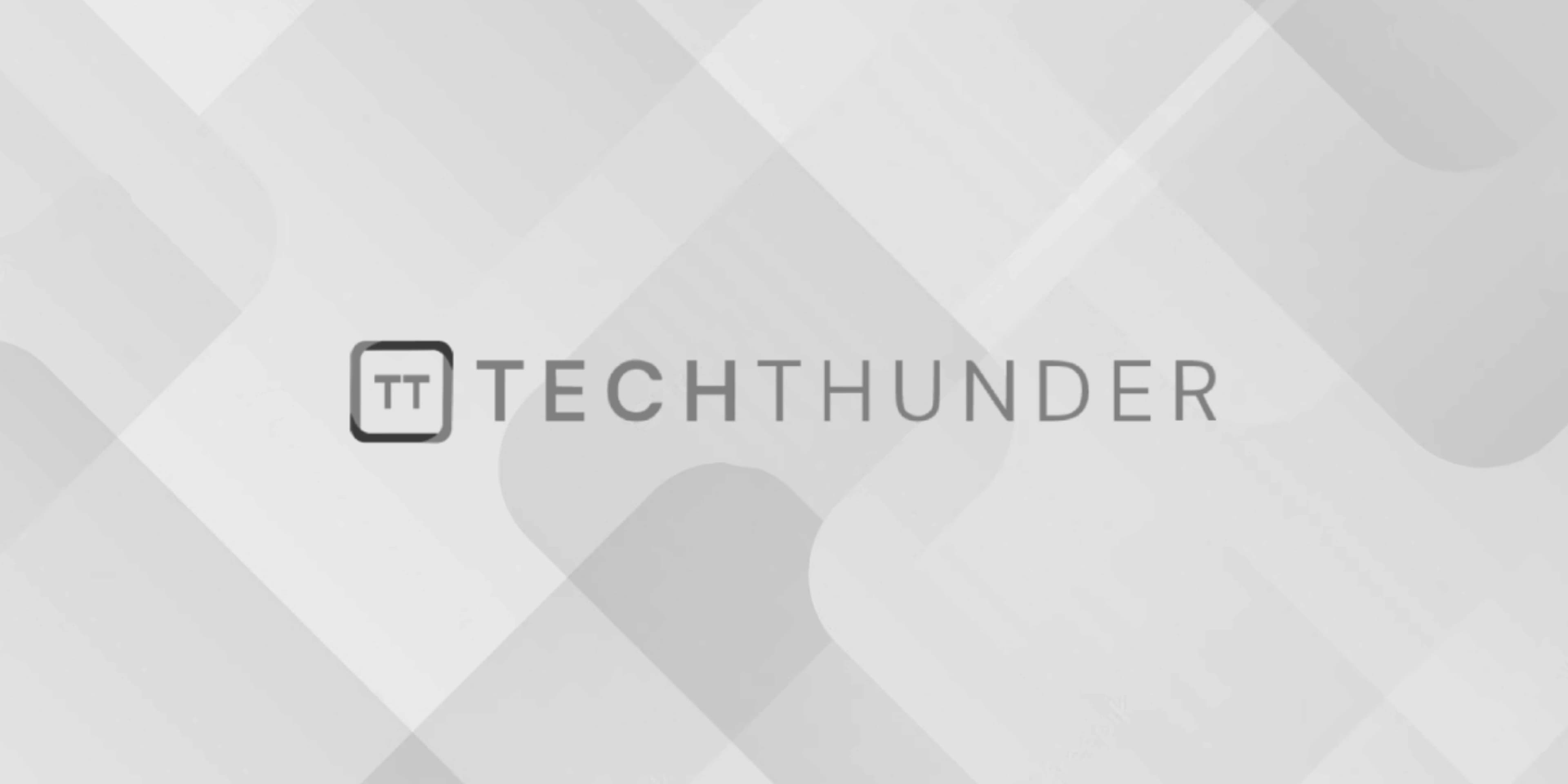
Copy elision in C++
Copy elision is an optimization technique in C++ that allows the compiler to avoid unnecessary copy or move operations when returning objects from functions or creating objects in certain situations. This optimization can significantly improve the performance of C++ programs by eliminating the need for redundant copy or move constructors.
Copy elision is defined by the C++ standard and is supported by most modern C++ compilers. It is also known as “return value optimization” (RVO) and “named return value optimization” (NRVO).
Here’s how copy elision works in C++:
- Return Value Optimization (RVO): When a function returns an object by value, and that object is constructed inside the function, the compiler can optimize the construction and return of the object. Instead of creating the object inside the function, copying or moving it to a temporary, and then returning the temporary, the compiler can construct the object directly in the caller’s scope, avoiding any unnecessary copy or move operations.
// Before copy elision (without RVO)
MyObject func() {
MyObject obj;
// ...
return obj;
}
// After copy elision (with RVO)
MyObject func() {
// ...
return MyObject(); // Construct directly in the caller's scope
}
- Named Return Value Optimization (NRVO): Similar to RVO, NRVO occurs when a function returns an object by value that is constructed inside the function. However, even if the object is given a name inside the function, the compiler can still optimize the construction and return of the object by eliminating unnecessary copies or moves.
// Before copy elision (without NRVO)
MyObject func() {
MyObject obj;
// ...
return obj; // Named object
}
// After copy elision (with NRVO)
MyObject func() {
// ...
MyObject obj; // Named object
return obj; // Object is still constructed directly in the caller's scope
}
Copy elision is transparent to the programmer and occurs automatically if the compiler determines that it can be applied safely. However, in some cases, copy elision may not be possible, such as when the copy or move constructor has side effects.
Copy elision can lead to improved performance by reducing unnecessary object construction, copying, and destruction. It is a standard-compliant optimization and is widely supported by C++ compilers.