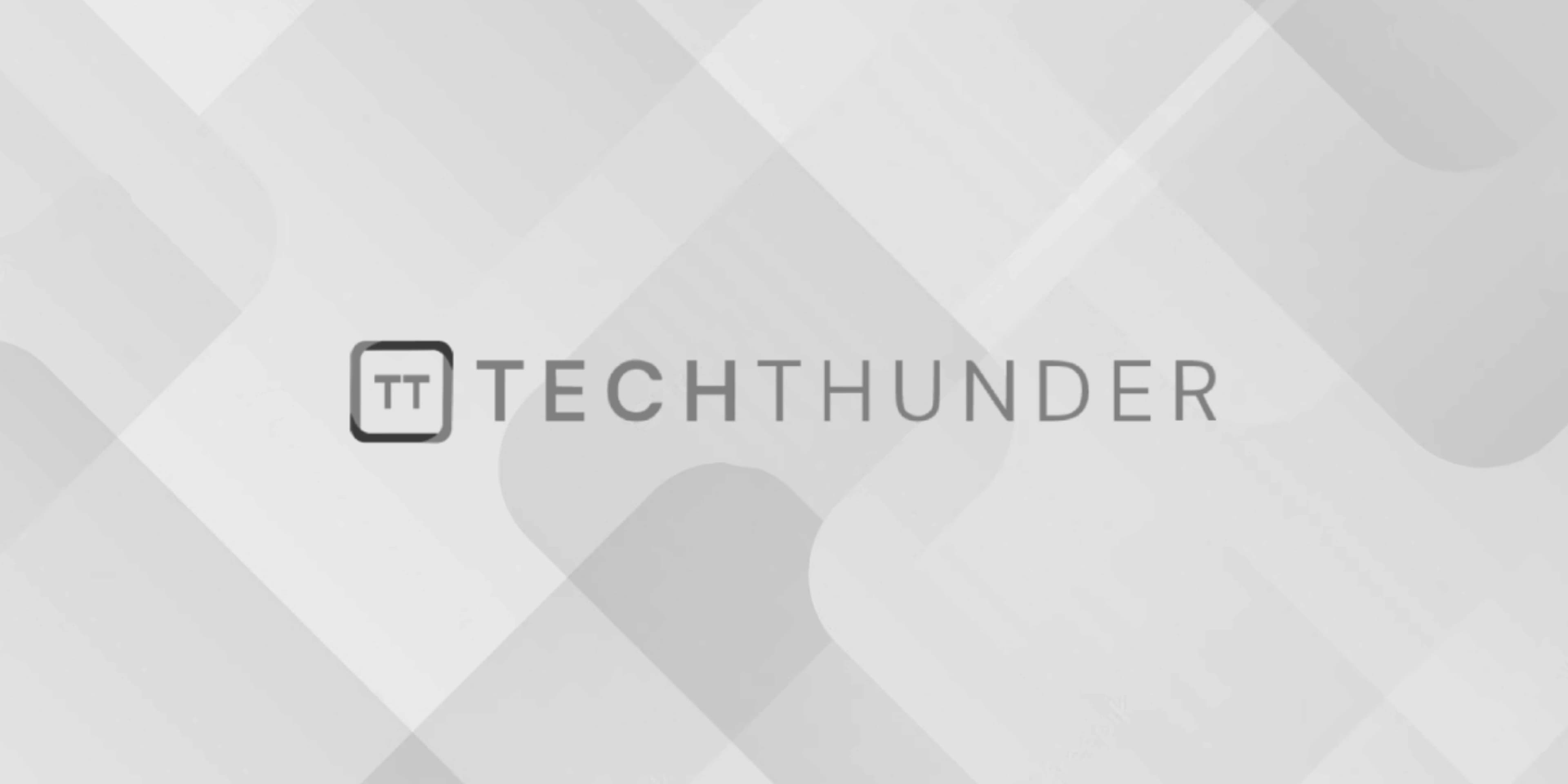
C++ Recursion
Recursion in C++ is a programming technique where a function calls itself to solve a problem. It’s a powerful and elegant way to solve problems that can be broken down into smaller, similar subproblems. Recursive functions have two parts: the base case(s) and the recursive case(s).
Here’s how recursive functions work in C++:
- Base Case(s): The base case(s) are one or more conditions that specify when the recursion should stop. When the base case is met, the recursive function stops calling itself and returns a value. The base case is crucial to prevent infinite recursion.
- Recursive Case(s): The recursive case(s) involve breaking down a larger problem into one or more smaller, similar subproblems. The function calls itself with modified arguments to solve these subproblems.
Here’s a simple example of a recursive function to calculate the factorial of a non-negative integer:
#include <iostream>
unsigned long long factorial(int n) {
// Base case: factorial of 0 or 1 is 1
if (n == 0 || n == 1) {
return 1;
}
// Recursive case: n! = n * (n-1)!
else {
return n * factorial(n - 1);
}
}
int main() {
int num = 5;
unsigned long long result = factorial(num);
std::cout << "Factorial of " << num << " is " << result << std::endl;
return 0;
}
In this example, the factorial
function has a base case for n
equal to 0 or 1, which returns 1. For other values of n
, it recursively calls itself with a smaller value (n-1
) and multiplies the result by n
.
Key points to remember when working with recursion in C++:
- Ensure there’s a well-defined base case to stop the recursion.
- Ensure that each recursive call reduces the problem towards the base case.
- Recursive functions can consume a lot of memory due to multiple function calls on the stack. Consider optimizing or using an iterative approach for problems with large input sizes.
- Tail recursion is a special type of recursion where the recursive call is the last operation in the function. Some compilers can optimize tail-recursive functions into efficient iterative code.
Here’s an example of a tail-recursive factorial function:
unsigned long long factorial_tail_recursive(int n, unsigned long long result = 1) {
if (n == 0 || n == 1) {
return result;
} else {
return factorial_tail_recursive(n - 1, n * result);
}
}
Recursion is a versatile technique that can be applied to various problems, including tree traversal, maze solving, and mathematical calculations. Understanding when and how to use recursion effectively is an essential skill for C++ programmers.