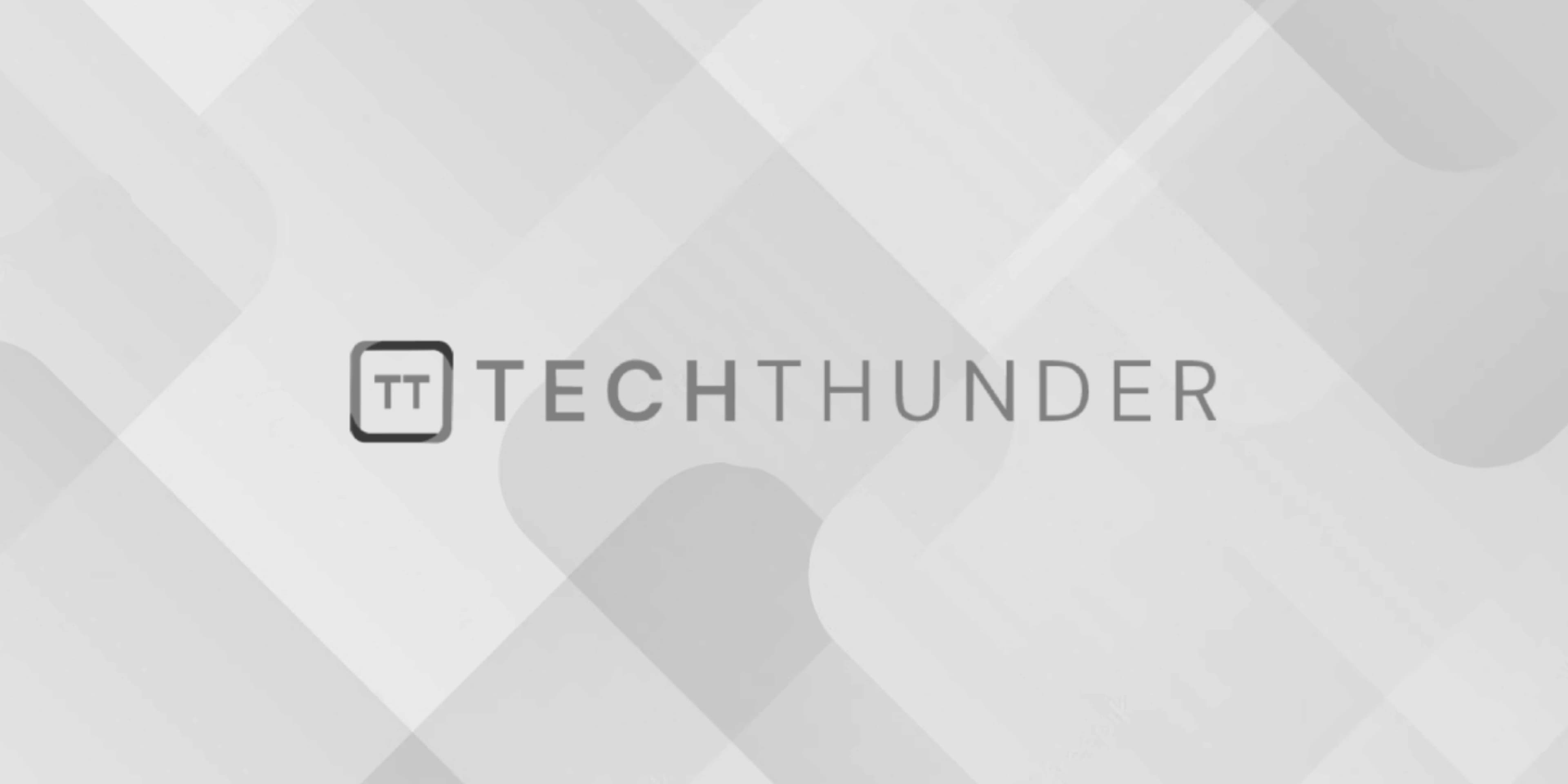
275 views
Array of sets in C++
The C++ can create an array of sets using the Standard Template Library (STL). This allows you to store multiple sets as elements of an array, where each set can hold a collection of unique values. Here’s how you can create and work with an array of sets:
C++
#include <iostream>
#include <set>
int main() {
const int arraySize = 3;
std::set<int> arrayOfSets[arraySize]; // Declare an array of sets
// Populate the sets
arrayOfSets[0].insert(1);
arrayOfSets[0].insert(2);
arrayOfSets[0].insert(3);
arrayOfSets[1].insert(3);
arrayOfSets[1].insert(4);
arrayOfSets[1].insert(5);
arrayOfSets[2].insert(5);
arrayOfSets[2].insert(6);
arrayOfSets[2].insert(7);
// Access and print the sets
for (int i = 0; i < arraySize; i++) {
std::cout << "Set " << i << ": ";
for (int value : arrayOfSets[i]) {
std::cout << value << " ";
}
std::cout << std::endl;
}
return 0;
}
In this code:
- We include the
<set>
header to use thestd::set
container from the STL. - We declare an array of sets named
arrayOfSets
with a specified size (arraySize
). - We populate each set in the array with unique integer values using the
insert
method. - We use a loop to iterate through the array of sets, printing the contents of each set.
This code demonstrates how to create and use an array of sets. You can access, modify, or manipulate each individual set in the array as needed for your specific application. Each set will automatically maintain its elements in a sorted and unique order.