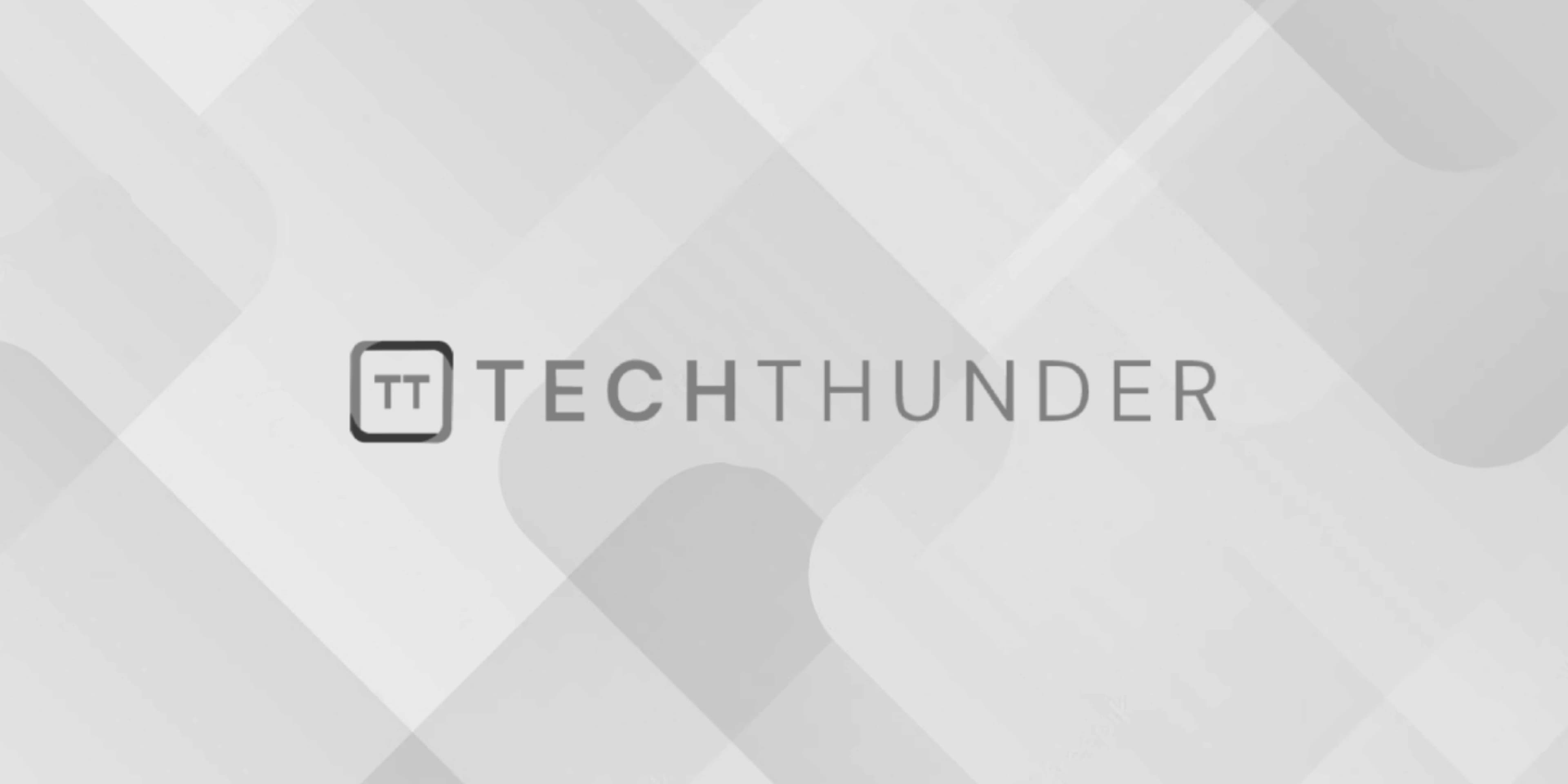
211 views
Default Virtual Behaviour in C++ and JAVA
The both C++ and Java, virtual functions/methods play a significant role in enabling polymorphism, where you can call the appropriate method of an object based on its runtime type, rather than its static type. However, there are some differences in the default behavior of virtual functions/methods in C++ and Java.
C++ Default Virtual Behavior:
- Virtual by Default: In C++, methods are not virtual by default. To make a function polymorphic, you need to explicitly declare it as virtual in the base class. This allows derived classes to override the function.
C++
class Base {
public:
virtual void foo() {
// Base class implementation
}
};
class Derived : public Base {
public:
void foo() override {
// Derived class implementation
}
};
- No Dynamic Binding Without
virtual
: If a function in the base class is not declared asvirtual
, then calls to that function are resolved at compile-time based on the static type of the object, not the runtime type. This is known as “early binding” or “static binding.” - Virtual Destructor: If a base class contains virtual functions, it’s a good practice to provide a virtual destructor in that class. This allows proper cleanup of derived class objects when deleting through a base class pointer.
Java Default Virtual Behavior:
- Virtual by Default: In Java, all non-static methods are implicitly virtual (or more accurately, dynamically bound) by default. You don’t need to use a keyword like
virtual
in Java.
C++
class Base {
void foo() {
// Base class implementation
}
}
class Derived extends Base {
void foo() {
// Derived class implementation
}
}
- Dynamic Binding Always: In Java, method calls are dynamically bound by default. The JVM determines the actual method to call at runtime based on the actual class of the object, not the reference type.
- No Concept of Destructor: Java doesn’t have a destructor concept like C++. Memory management in Java is automatic, handled by the garbage collector, so there’s no need to provide a destructor.
In summary, in C++, virtual behavior must be explicitly specified by marking a function as virtual
in the base class, and non-virtual functions are statically bound. In contrast, in Java, all methods are virtual by default, and dynamic binding always occurs. This default behavior reflects the different design philosophies and memory management models of the two languages.