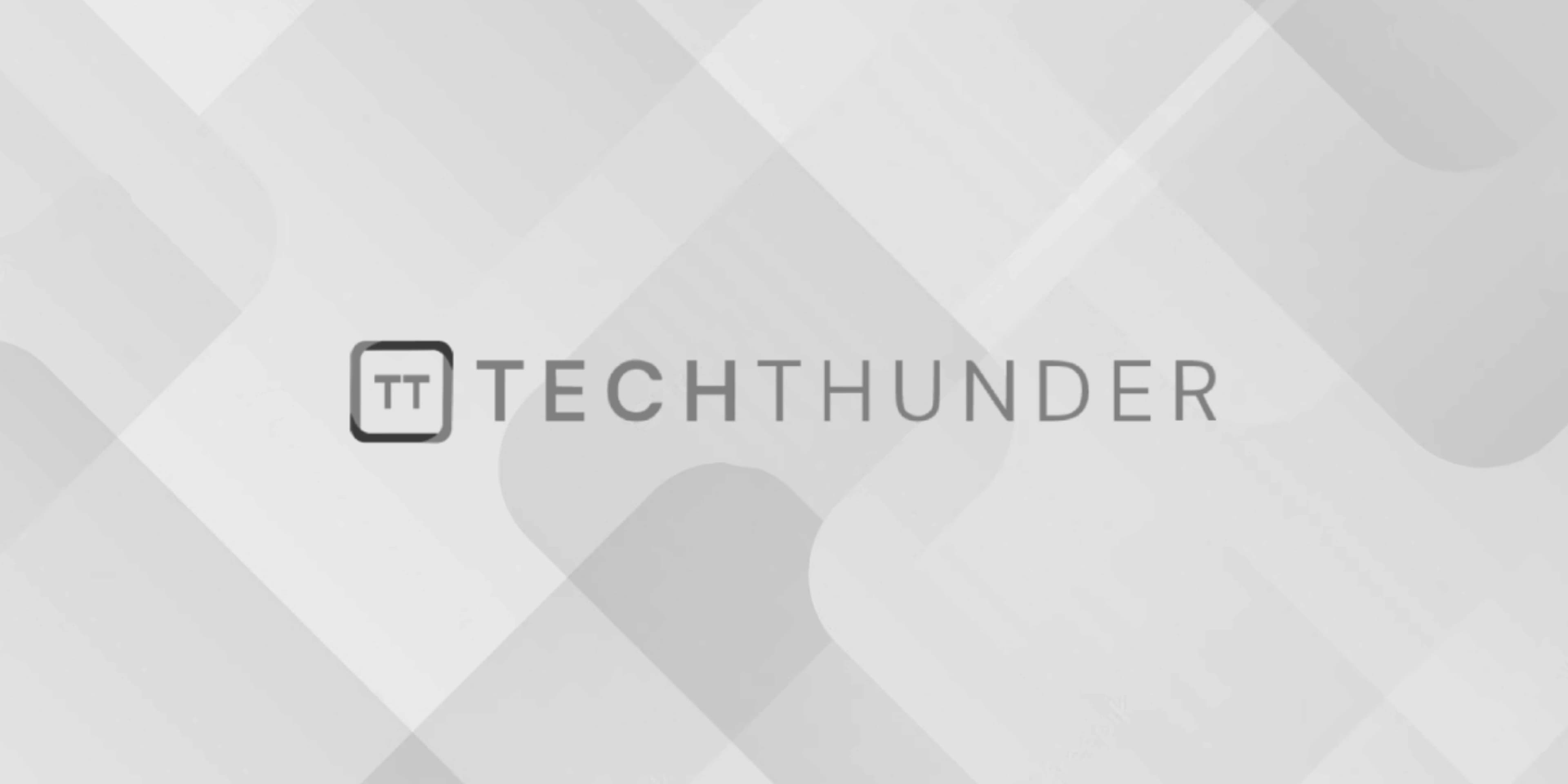
199 views
What is a reference variable in C++
The C++ reference variable is an alias or an alternative name for an existing object or variable. It allows you to access and manipulate the underlying data of the referenced object through the reference variable itself. References are often used to create more readable and efficient code.
Here’s how you declare and use a reference variable in C++:
C++
// Syntax for declaring a reference variable:
type &reference_variable = existing_variable;
// Example:
int x = 5;
int &ref = x; // ref is a reference to the variable x
// Now, you can use ref just like x:
ref = 10; // This changes the value of x to 10
// Any changes made to ref affect x, and vice versa.
Key points about reference variables in C++:
- They must be initialized when declared. You cannot have a reference without an initial value.
- Once a reference is bound to a variable, it cannot be rebound to another variable. It remains an alias for the initial variable.
- They are often used as function parameters to pass variables by reference, allowing functions to modify the original data.
- References are commonly used to avoid unnecessary copying of large objects, which can improve performance.
Here’s an example of using a reference as a function parameter:
C++
void modifyValue(int &ref) {
ref = 20;
}
int main() {
int x = 10;
modifyValue(x); // Pass x by reference
cout << x; // Output will be 20, as the function modified the original variable
return 0;
}
In this example, modifyValue
takes an integer reference as a parameter, and any changes made to ref
inside the function affect the original variable x
.