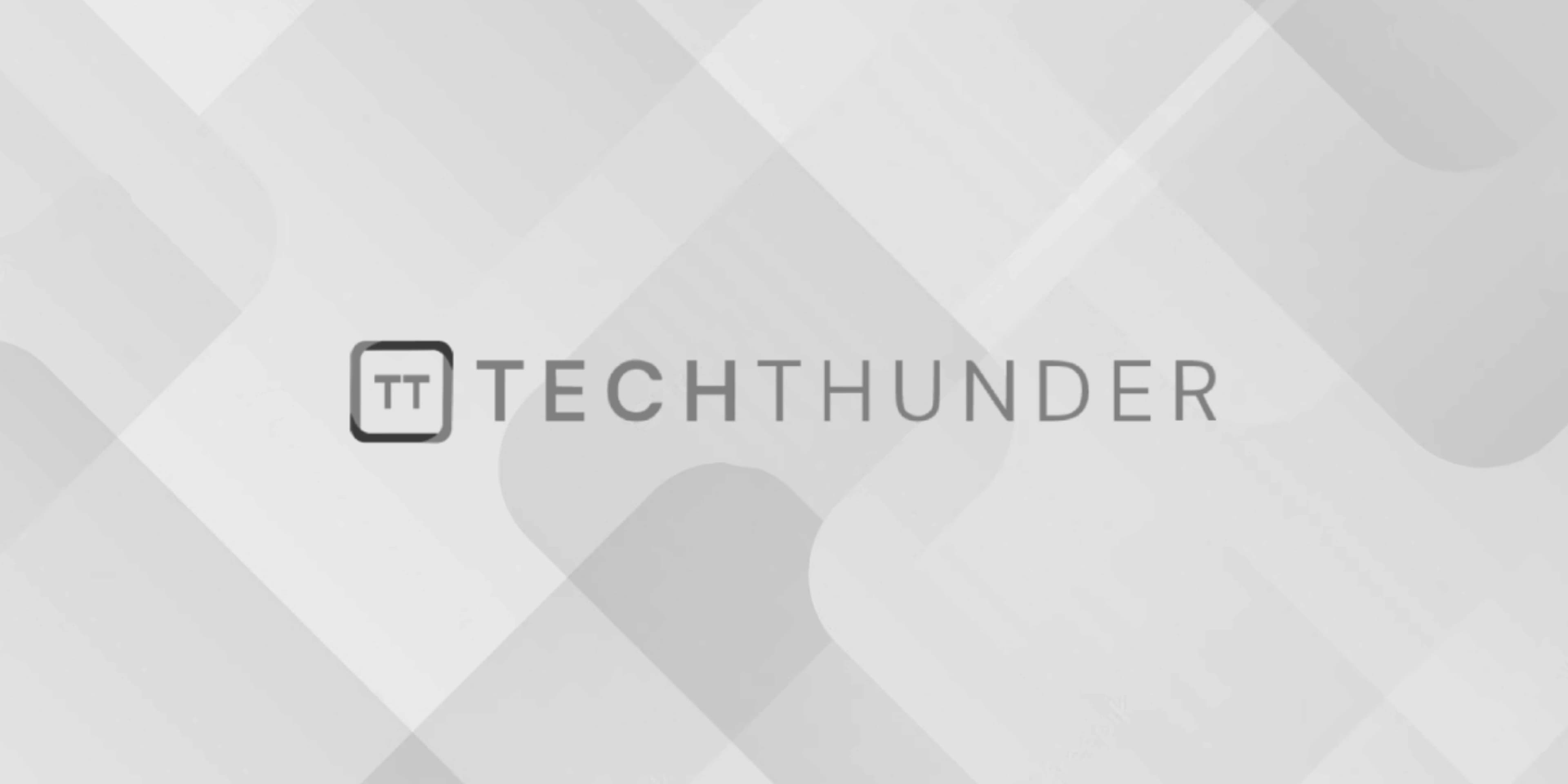
C++ Algorithm
C++ is a powerful programming language that provides a wide range of algorithms and data structures through the Standard Template Library (STL). The STL includes a collection of header files, such as <algorithm>
, <vector>
, <list>
, <map>
, and more, that provide various functions and classes for common programming tasks. Here’s an overview of some common algorithms and how to use them in C++:
Sorting Algorithms:
C++ provides several sorting algorithms, including std::sort
, std::stable_sort
, and std::partial_sort
, to sort arrays, vectors, and other containers.
#include <iostream>
#include <vector>
#include <algorithm>
int main() {
std::vector<int> numbers = {5, 2, 9, 1, 5};
// Sort the vector in ascending order
std::sort(numbers.begin(), numbers.end());
// Display the sorted vector
for (int num : numbers) {
std::cout << num << " ";
}
std::cout << std::endl;
return 0;
}
Searching Algorithms:
You can use std::find
, std::binary_search
, and other functions to search for elements in containers.
#include <iostream>
#include <vector>
#include <algorithm>
int main() {
std::vector<int> numbers = {5, 2, 9, 1, 5};
int target = 9;
// Search for the target element in the vector
auto result = std::find(numbers.begin(), numbers.end(), target);
if (result != numbers.end()) {
std::cout << "Found " << target << " at position " << std::distance(numbers.begin(), result) << std::endl;
} else {
std::cout << target << " not found in the vector." << std::endl;
}
return 0;
}
Other Algorithms:
C++ also offers a wide range of other algorithms for tasks like finding the minimum or maximum element, reversing containers, performing transformations, and more. You can explore these functions in the <algorithm>
header.
Custom Comparators:
Many algorithms allow you to specify custom comparison functions to customize how elements are compared.
#include <iostream>
#include <vector>
#include <algorithm>
bool customComparator(int a, int b) {
return a > b; // Sort in descending order
}
int main() {
std::vector<int> numbers = {5, 2, 9, 1, 5};
// Sort the vector in descending order using a custom comparator
std::sort(numbers.begin(), numbers.end(), customComparator);
// Display the sorted vector
for (int num : numbers) {
std::cout << num << " ";
}
std::cout << std::endl;
return 0;
}
These are just a few examples of the algorithms available in C++ through the STL. To use these algorithms effectively, include the necessary headers and familiarize yourself with the documentation for the functions and containers you plan to use. The C++ Standard Library provides a rich set of tools to simplify common programming tasks.