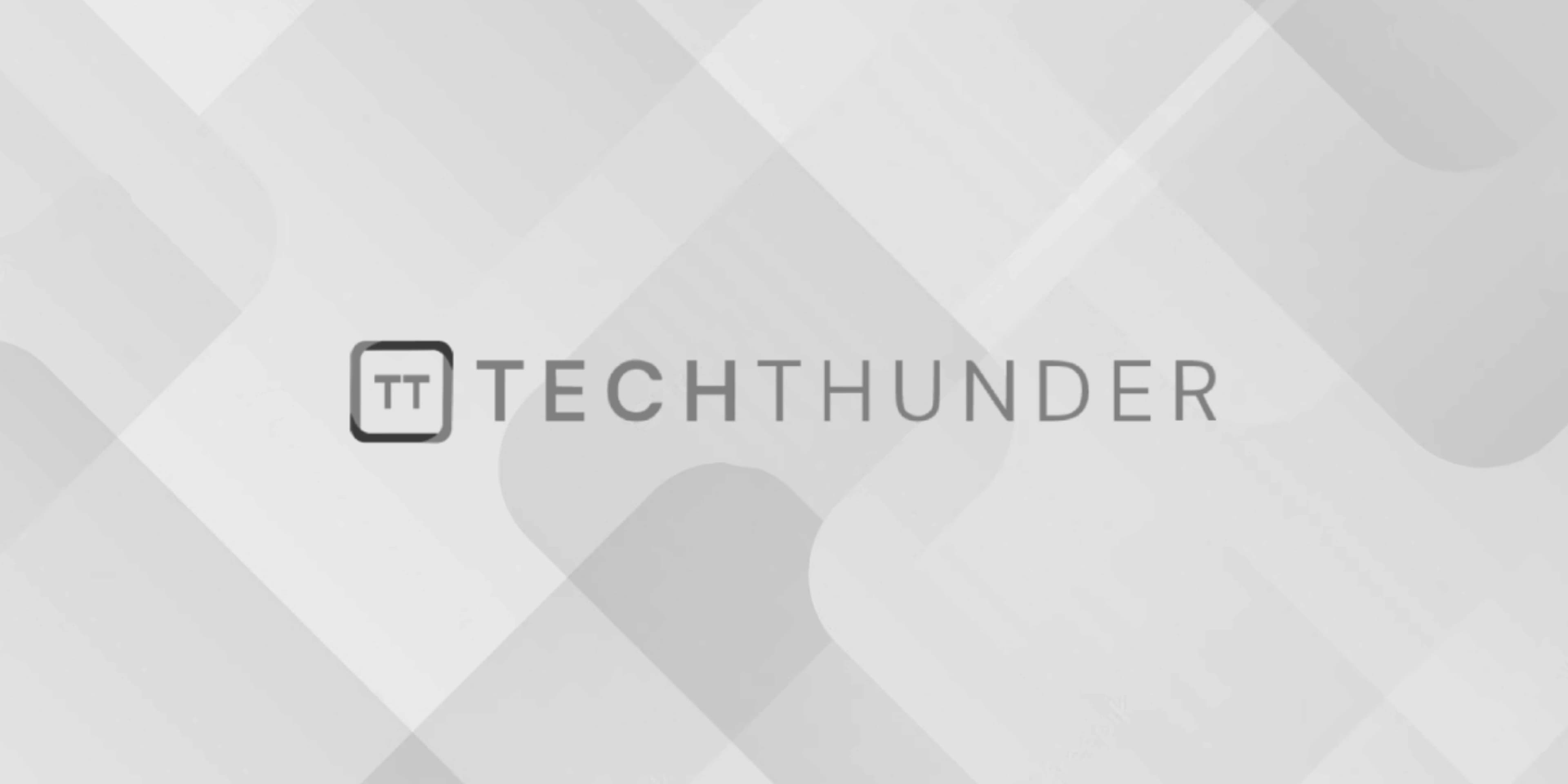
213 views
C++ Strings
The C++ strings are sequences of characters represented using the std::string
class from the Standard Library. C++ provides a convenient and powerful way to work with strings using the std::string
class, which is part of the Standard Template Library (STL).
Here are some common operations and features related to C++ strings:
- String Declaration and Initialization: You can declare and initialize strings using various methods:
C++
std::string str1 = "Hello, World!";
std::string str2("C++ Strings");
- String Concatenation: You can concatenate strings using the
+
operator or theappend()
member function:
C++
std::string result = str1 + " " + str2;
str1.append(" and C++ are fun!");
- String Length: You can find the length of a string using the
length()
orsize()
member functions:
C++
int len = str1.length();
- Accessing Characters: Individual characters in a string can be accessed using the
[]
operator or theat()
member function:
C++
char firstChar = str1[0];
char secondChar = str1.at(1);
- Substrings: You can extract substrings from a string using the
substr()
member function:
C++
std::string sub = str1.substr(0, 5); // Extracts "Hello" from str1
- String Comparison: You can compare strings using comparison operators (
==
,!=
,<
,<=
,>
,>=
) or thecompare()
member function:
C++
if (str1 == str2) {
// Strings are equal
}
- Searching and Finding: You can search for substrings within a string using functions like
find()
andrfind()
:
C++
size_t found = str1.find("World"); // Returns the position of "World" in str1
- String Modification: You can modify a string by replacing substrings using functions like
replace()
:
C++
str1.replace(7, 5, "Universe"); // Replaces "World" with "Universe" in str1
- String Input and Output: You can read and write strings using standard input and output streams:
C++
std::cout << "Enter your name: ";
std::cin >> str1;
- String Iteration: You can iterate over the characters in a string using loops:
for (char c : str1) { std::cout << c << " "; }
- String Manipulation: C++ provides several functions in the
<cstring>
header for string manipulation, such asstrcpy()
,strcat()
,strlen()
, and more. However, it’s often more convenient and safer to usestd::string
for most string operations.
C++ strings (std::string
) provide dynamic memory management, automatic resizing, and a wide range of member functions, making them a versatile choice for working with text data. They handle memory allocation and deallocation, which simplifies string manipulation and reduces the risk of memory-related bugs.