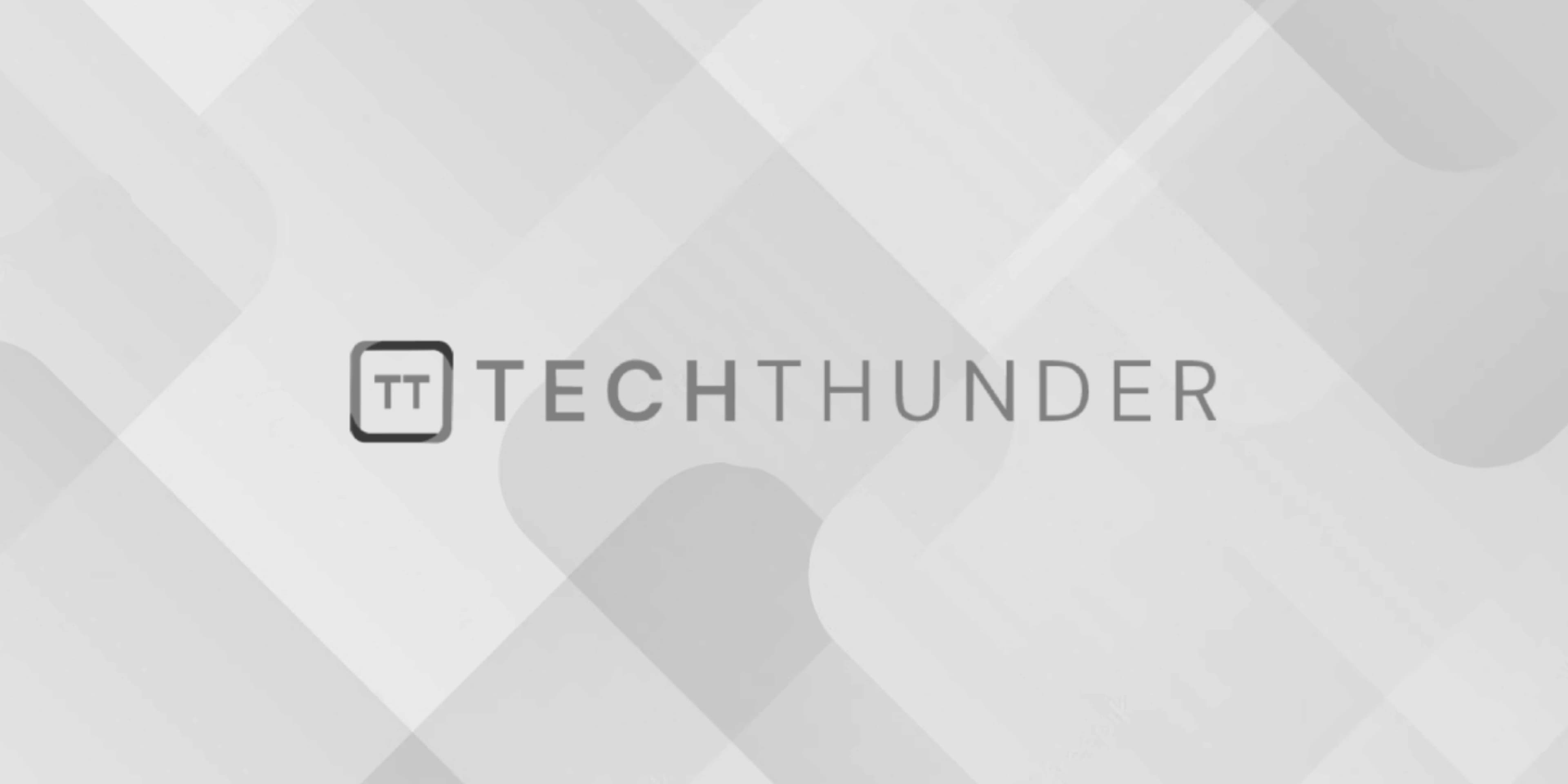
C++ if-else
The C++ if-else
statement is used to control the flow of a program based on a condition. It allows you to execute different blocks of code depending on whether the condition evaluates to true
or false
. The basic syntax of the if-else
statement is as follows:
if (condition) {
// Code to execute if the condition is true
} else {
// Code to execute if the condition is false
}
Here’s an example of how to use if-else
:
#include <iostream>
int main() {
int num;
std::cout << "Enter an integer: ";
std::cin >> num;
if (num > 0) {
std::cout << "The number is positive." << std::endl;
} else if (num < 0) {
std::cout << "The number is negative." << std::endl;
} else {
std::cout << "The number is zero." << std::endl;
}
return 0;
}
In this example:
- The user is prompted to enter an integer.
- The program uses the
if-else
statement to check whether the entered number is positive, negative, or zero. - Depending on the condition, the program executes the appropriate block of code.
The else if
part allows you to check additional conditions if the initial if
condition is false. The else
part provides a fallback block of code to execute when none of the conditions are true.
You can also use the if-else
statement without the else if
or else
parts, depending on your specific needs. For example:
if (condition) {
// Code to execute if the condition is true
}
You can also nest if-else
statements within each other to create more complex decision structures. However, be cautious with nested if-else
statements, as they can become hard to read and understand if overused.
if (condition1) {
// Code for condition1
if (condition2) {
// Code for condition2
} else {
// Code if condition2 is false
}
} else {
// Code for condition1 is false
}
The if-else
statement is a fundamental control structure in C++ and is essential for implementing conditional logic in your programs.